A Beginner’s Guide to PHP for Sngine Customizations
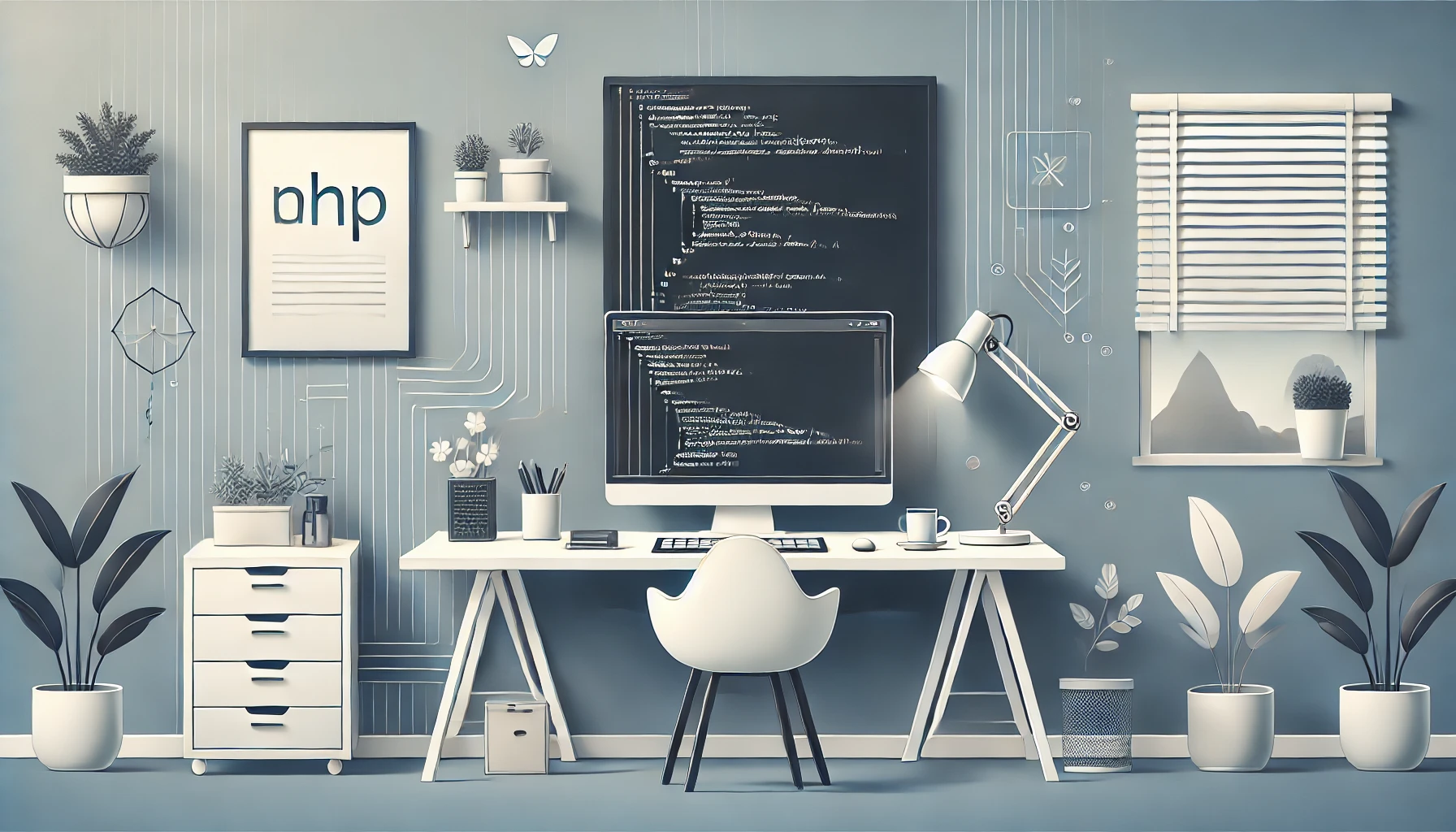
Learn PHP basics relevant to Sngine, like variables, loops, and arrays.
Introduction
Sngine is a dynamic and customizable social networking platform powered by PHP. For webmasters, understanding PHP opens the doors to endless possibilities for personalizing their Sngine community. This guide provides a comprehensive walkthrough of PHP basics tailored for Sngine users, enabling them to make meaningful changes while adhering to the platform's core structure.
Whether you're a beginner or have some coding knowledge, this article will help you understand PHP's role in Sngine, how to write efficient code, and practical ways to integrate new functionalities into your platform.
Why PHP Is Essential for Sngine
Sngine's backbone is PHP, making it the primary tool for customization and development. Here's why you need to learn PHP:
- Core Logic: Sngine's features, from user authentication to content rendering, are PHP-driven.
- Dynamic Content: PHP powers interactive elements like newsfeeds, messaging systems, and notifications.
- Custom Modules: Adding plugins or custom features requires PHP expertise.
- Integration: Connect APIs, external services, or databases with PHP for enhanced functionalities.
By mastering PHP basics, you'll unlock the full potential of Sngine, enabling you to create a platform that stands out.
Setting Up Your Environment
Before diving into PHP, set up the right tools to ensure a seamless coding experience:
1. Install XAMPP or WampServer
Local servers like XAMPP or WampServer provide the environment needed to run PHP scripts. Here's how to set them up:
- Download and install XAMPP/WampServer from their official websites.
- Place your Sngine project files in the
htdocs
(XAMPP) orwww
(WampServer) directory. - Start the server and access your project at
http://localhost/project-folder
.
2. Choose a Code Editor
Select an editor that supports syntax highlighting and debugging for PHP. Popular choices include:
- Visual Studio Code (free, extensible, and beginner-friendly).
- PHPStorm (advanced features for PHP development).
3. Set Up Sngine Locally
Install Sngine in your local server environment for testing:
- Extract Sngine files into the appropriate directory.
- Create a database using PHPMyAdmin and configure the
config.php
file to connect Sngine to this database.
Understanding PHP Basics
Let’s dive into PHP fundamentals with examples relevant to Sngine customizations.
1. PHP Syntax
PHP scripts start with <?php
and end with ?>
. For example:
<?php
echo "Hello, Sngine!";
?>
This script outputs: Hello, Sngine!
2. Variables in PHP
Variables are used to store data. In PHP, they start with $
.
Example:
<?php
$site_name = "Sngine";
echo "Welcome to $site_name!";
?>
Output: Welcome to Sngine!
3. Conditional Statements
Control the flow of your code with conditional statements like if
, else
, and elseif
.
Example: Displaying User Roles
<?php
$user_role = "admin";
if ($user_role == "admin") {
echo "Welcome, Admin!";
} elseif ($user_role == "moderator") {
echo "Hello, Moderator!";
} else {
echo "Access Denied.";
}
?>
4. PHP Loops
Loops allow you to execute a block of code multiple times.
For Loop
<?php
for ($i = 1; $i <= 5; $i++) {
echo "User $i <br>";
}
?>
While Loop
<?php
$i = 1;
while ($i <= 3) {
echo "Processing user $i <br>";
$i++;
}
?>
Foreach Loop
Useful for working with arrays.
<?php
$users = array("Alice", "Bob", "Charlie");
foreach ($users as $user) {
echo "User: $user <br>";
}
?>
5. Arrays in PHP
Arrays store multiple values in a single variable.
Indexed Arrays
<?php
$users = array("Alice", "Bob", "Charlie");
echo $users[0]; // Outputs: Alice
?>
Associative Arrays
<?php
$user = array("name" => "Alice", "role" => "admin");
echo $user['name']; // Outputs: Alice
?>
6. PHP Functions
Functions encapsulate reusable blocks of code.
Example: Greeting Function
<?php
function greet_user($name) {
return "Hello, $name!";
}
echo greet_user("Sngine User");
?>
Customizing Sngine Using PHP
1. Adding Custom Variables
To display a custom message on the homepage:
- Open
index.php
. - Add:
phpCopy code
$custom_message = "Welcome to My Sngine!";
- Assign it to Smarty:
phpCopy code
$smarty->assign('custom_message', $custom_message);
- Display it in the
.tpl
file:htmlCopy code<p>{$custom_message}</p>
2. Fetching Data from the Database
Example: Display all users.
<?php
$query = "SELECT * FROM users";
$result = $db->query($query);
while ($user = $result->fetch_assoc()) {
echo "User: " . $user['user_name'] . "<br>";
}
?>
3. Creating a Custom Plugin
Add a feature to display user birthdays:
- Open
includes/class-user.php
and add:phpCopy codepublic function get_user_birthdays() { global $db; $query = "SELECT * FROM users WHERE MONTH(user_birthdate) = MONTH(CURDATE())"; return $db->query($query)->fetch_all(MYSQLI_ASSOC); }
- Assign it to Smarty in
index.php
:phpCopy code$birthdays = $user->get_user_birthdays(); $smarty->assign('birthdays', $birthdays);
- Display in the
.tpl
file:htmlCopy code{foreach from=$birthdays item=birthday} <p>{$birthday.user_name}</p> {/foreach}
Best Practices
- Follow Sngine’s Style: Maintain consistency with its existing structure.
- Secure Your Code: Validate and sanitize inputs to prevent vulnerabilities.
- Document Changes: Add comments for better maintainability.
Conclusion
By mastering PHP basics, you'll gain the skills needed to customize Sngine to suit your community’s unique needs. Use this guide as a foundation, and remember to experiment and build on your knowledge. The possibilities with Sngine and PHP are endless.
- Cómo empezar
- Customization and Themes
- Plugins and Extensions
- SEO and Marketing
- Web Hosting and Performance
- Monetization and Business
- Community Building
- E-commerce and Marketplace
- Security and Privacy
- Development and Coding
- Bug Reports and Fixes
- Hosting Reviews
- Success Stories
- FAQs and Guides
- Feature Requests
- Social Media Integration
- Event Management
- Analytics and Reporting
- Collaborative Projects
- Sngine Updates and News
- Theater
- Wellness
