Using Smarty Templates in Sngine: A Comprehensive Guide
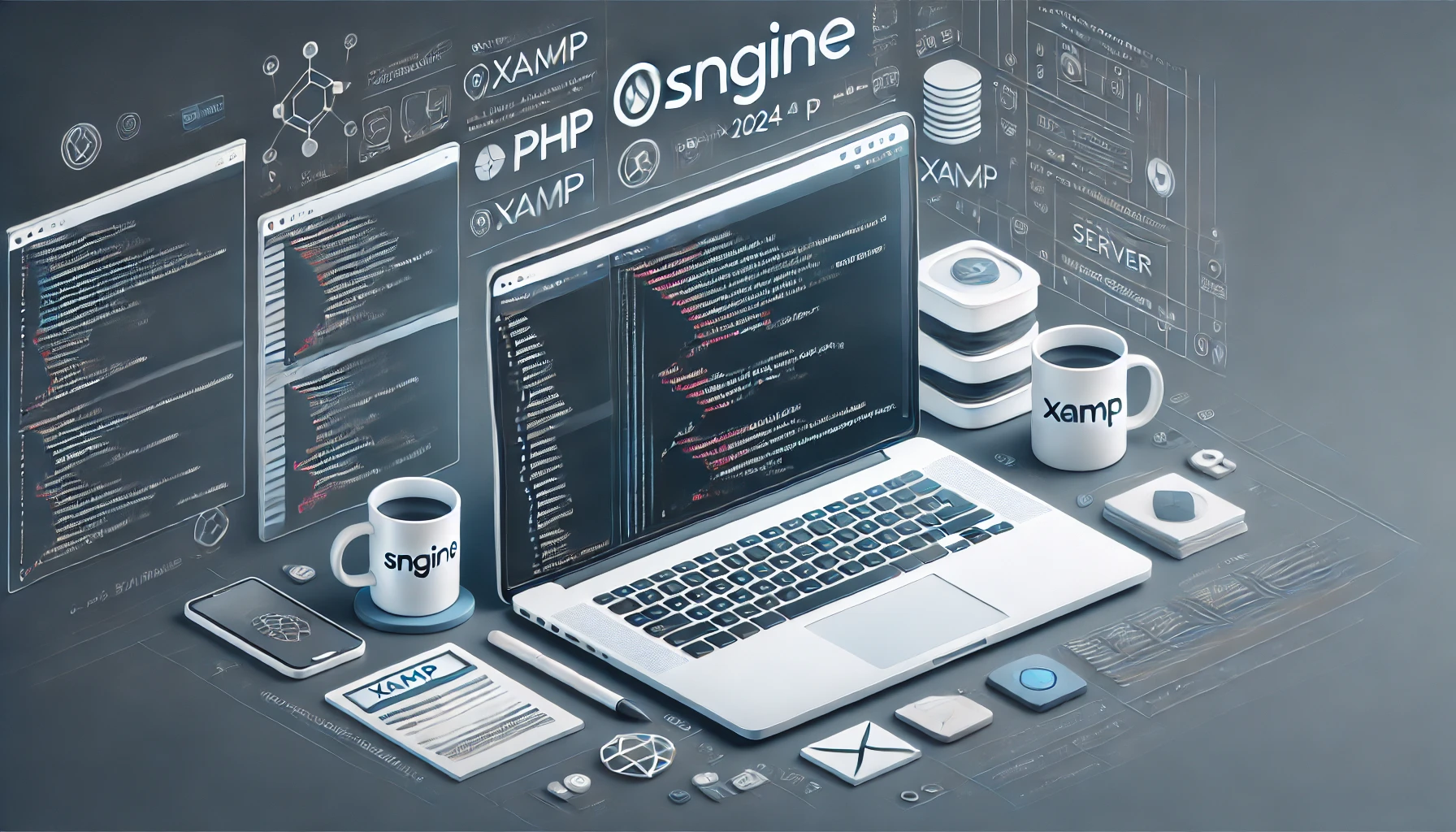
How Sngine Leverages Smarty for Templating and How You Can Modify Templates Efficiently
Sngine, a flexible social networking script, is powered by Smarty—a PHP-based templating engine that simplifies the development process by separating business logic from presentation. This guide provides a detailed walkthrough of Smarty templates, their significance in Sngine, and how you can effectively customize them to meet your specific requirements.
What is Smarty?
Smarty is a widely-used PHP templating engine that bridges back-end functionality with front-end design. By isolating PHP logic from HTML, it streamlines development, enhances maintainability, and allows for a clear focus on the user interface. Smarty’s features include:
- Template Caching: Optimizes performance by caching compiled templates.
- Reusable Components: Encourages modular design for easier updates.
- Powerful Syntax: Offers loops, conditionals, functions, and plugins for dynamic content rendering.
- Separation of Concerns: Keeps logic out of templates, ensuring clean and manageable codebases.
Sngine’s File Structure and Smarty Templates
In Sngine, Smarty templates reside in the content/themes/
directory under your active theme. Key files include:
_head.tpl
: Loads stylesheets, metadata, and JavaScript files._sidebar.tpl
: Manages sidebar widgets and additional content.index.tpl
: Defines the homepage structure and layout._footer.tpl
: Handles the footer content and scripts.- Widgets: Located in the
widgets/
folder, these templates offer modular features like user stats, birthdays, or advertisements.
Understanding Smarty Syntax
1. Variables
Smarty variables are represented within curly braces:
{$variable_name}
Example:
<p>Hello, {$user_name}!</p>
2. Loops
Smarty’s foreach
loop is used to iterate over arrays:
{foreach from=$posts item=post}
<h2>{$post.title}</h2>
{/foreach}
3. Conditional Statements
Use if
, else
, and elseif
for conditional rendering:
{if $user_logged_in}
<p>Welcome, {$user_name}!</p>
{else}
<p>Please log in to continue.</p>
{/if}
4. Functions
Smarty includes functions like include
, assign
, and fetch
to enable reusable components:
{include file="widgets/my_widget.tpl"}
How to Modify Smarty Templates in Sngine
Step 1: Locate the Template Files
Navigate to your active theme’s templates
folder. For example:
content/themes/default/templates/
Step 2: Backup the Files
Before editing, always back up your original template files. This precaution ensures that you can restore functionality if changes lead to unexpected issues.
Step 3: Add or Modify Widgets
To create a custom widget:
- Create a Widget File:
Create a new file in thewidgets/
folder, e.g.,my_custom_widget.tpl
.smartyCopy code<div class="custom-widget"> <h3>Custom Widget Title</h3> <p>This is a custom widget.</p> </div>
- Include the Widget:
Add the following line to your_sidebar.tpl
or another suitable template:smartyCopy code{include file="widgets/my_custom_widget.tpl"}
Step 4: Test Your Changes
After saving your modifications, test them in your local environment to ensure proper functionality.
Creating Custom Templates
1. Customizing the Homepage
Let’s add a banner to the homepage:
- Step 1: Open
index.tpl
in your theme’stemplates/
folder. - Step 2: Insert the following code snippet where you want the banner to appear:
smartyCopy code
<div class="homepage-banner"> <img src="{$system['system_url']}/content/themes/{$system['theme']}/images/banner.jpg" alt="Banner"> </div>
- Step 3: Save your changes and upload the banner image to the
images/
folder.
2. Adding a Dynamic Footer Section
- Open
_footer.tpl
. - Add the following code:
smartyCopy code
<div class="dynamic-footer"> <p>Powered by Sngine. All rights reserved.</p> {if $user_logged_in} <p>Logged in as: {$user_name}</p> {/if} </div>
Advanced Customizations
Using Smarty Plugins in Sngine
Smarty plugins extend the functionality of the template engine.
- Create a Modifier Plugin:
Navigate to theincludes/plugins/
directory and create a new PHP file, e.g.,modifier.capitalize.php
.phpCopy codefunction smarty_modifier_capitalize($string) { return ucwords($string); }
- Apply the Modifier in Templates:
smartyCopy code
{$title|capitalize}
Dynamic Variables from PHP
Assign dynamic content to templates using PHP:
$smarty->assign('user_notifications', $notifications);
Access these variables in the template:
{foreach from=$user_notifications item=notification}
<p>{$notification.text}</p>
{/foreach}
To be continued...
Continuing: Using Smarty Templates in Sngine
Best Practices for Using Smarty in Sngine
-
Keep Logic in PHP, Presentation in Templates
Minimize business logic in Smarty templates. Use PHP for calculations and pass only the required data to the templates. -
Use Reusable Components
Break your templates into smaller, reusable components (widgets). For instance, create auser_profile_widget.tpl
for user profile information that can be included across multiple pages. -
Clear Cache After Changes
Smarty caches templates for faster performance. When you make template changes, clear the cache by deleting the contents of thecontent/cache/
folder or using Sngine’s admin tools. -
Comment Your Code
Add comments to templates to explain customizations. This helps maintain clarity for future developers:smartyCopy code{* Custom sidebar widget for displaying user statistics *}
Real-World Examples of Smarty in Sngine
1. Adding a “Popular Posts” Widget
To display the most popular posts:
- Create a new widget file in
widgets/
namedpopular_posts.tpl
. - Add the following code:
smartyCopy code
<div class="popular-posts-widget"> <h3>Popular Posts</h3> {foreach from=$popular_posts item=post} <p><a href="{$system['system_url']}/posts/{$post.id}">{$post.title}</a></p> {/foreach} </div>
- Assign
$popular_posts
in the relevant PHP controller:phpCopy code$smarty->assign('popular_posts', $db->query("SELECT id, title FROM posts ORDER BY views DESC LIMIT 5"));
- Include the widget in
_sidebar.tpl
:smartyCopy code{include file="widgets/popular_posts.tpl"}
2. Custom Error Pages
To create a custom error page for 404
errors:
- Edit
404.tpl
in thetemplates/
folder. - Add the following code:
smartyCopy code
<div class="error-page"> <h1>404: Page Not Found</h1> <p>Sorry, the page you are looking for doesn’t exist.</p> </div>
Troubleshooting Smarty Templates
1. Templates Not Loading
- Ensure that file paths are correct in
include
statements. - Verify that the correct theme is active in the admin panel.
2. Variable Errors
If a variable is not defined, confirm it is assigned in the corresponding PHP file. Example:
$smarty->assign('user_name', $user_name);
3. Styling Issues
Check that your custom CSS is properly linked in _head.tpl
:
<link rel="stylesheet" href="{$system['system_url']}/content/themes/{$system['theme']}/css/custom.css">
Example Use Case: Adding a Birthday Notification
1. Create the Widget File
In widgets/
, create birthday_notification.tpl
:
<div class="birthday-notification">
{if $friends_birthday}
<p>Today’s Birthday: {$friends_birthday.name}</p>
{/if}
</div>
2. Add the Functionality in PHP
Update the PHP logic to fetch friend birthdays:
$today = date('Y-m-d');
$query = "SELECT * FROM users WHERE birthdate = '$today'";
$result = $db->query($query);
$friends_birthday = $result->fetch_assoc();
$smarty->assign('friends_birthday', $friends_birthday);
3. Include the Widget
Include the widget in _sidebar.tpl
:
{include file="widgets/birthday_notification.tpl"}
Conclusion
Smarty templates provide a robust and efficient way to customize and extend Sngine. By understanding Smarty syntax, Sngine’s file structure, and best practices, webmasters can create unique, user-friendly platforms that engage their audiences. With reusable components, clean code, and modular designs, your Sngine site can evolve into a thriving online community tailored to your vision.
This guide equips you with the foundational skills to start working with Smarty in Sngine effectively. As you explore further, you’ll find endless possibilities to enhance and customize your platform.
- Начало работы
- Customization and Themes
- Plugins and Extensions
- SEO and Marketing
- Web Hosting and Performance
- Monetization and Business
- Community Building
- E-commerce and Marketplace
- Security and Privacy
- Development and Coding
- Bug Reports and Fixes
- Hosting Reviews
- Success Stories
- FAQs and Guides
- Feature Requests
- Social Media Integration
- Event Management
- Analytics and Reporting
- Collaborative Projects
- Sngine Updates and News
- Theater
- Wellness
